A*
A* (pronounced "A-star") is a graph traversal and path search algorithm that uses heuristics to estimate the most efficient path.
As it traverses the graph, A* estimates the total cost of a path using each node on the way to the target.
The best path is calculated by minimizing the function \(f(n)=g(n)+h(n)\), where:
-
\(n\) is the next node on the path
-
\(g(n)\) is the cost of the path from the source node to \(n\)
-
\(h(n)\) is a heuristic function that estimates the cost of the cheapest path from \(n\) to the target node.
The algorithm terminates when the path it chooses to extend is a path from start to goal or if there are no paths eligible to be extended. The heuristic function is problem-specific. If the heuristic function is admissible, meaning that it never overestimates the actual cost to get to the target, the algorithm is guaranteed to return a least-cost path from source to target.
Specifications
CREATE QUERY tg_astar(VERTEX source_vertex, VERTEX target_vertex, SET<STRING> e_type, STRING wt_type,
STRING wt_attr, BOOL display = False)
Parameters
Parameter | Description | Default value |
---|---|---|
|
ID of the source vertex |
(empty string) |
|
ID of the target vertex |
(empty string) |
|
Edge types to traverse |
(empty string) |
|
The data type of the edge weights, if applicable. (Must be one of |
(empty string) |
|
One of a pair of attributes used to calculate distance. |
(empty string) |
|
One of a pair of attributes used to calculate distance. |
(empty string) |
|
The attribute used as edge weight, if applicable |
(empty string) |
|
Whether to output edges for visualization |
True |
Example
We run the tg_astar
algorithm to find the shortest path between the source vertex "Kings Cross" and the target vertex "Kentish Town" on a graph which is a transport network of stations.
Each station has its geometric attibutes(i.e.latitude and longitude) and the edge weight represents the distance between stations in kilometers.
The heuristic function used to guide the search is the Haversine Formula, which computes the distance between two points on a sphere given their longitudes and latitudes.
# Use _ for default values
RUN QUERY astar(("Kings Cross","Station"), ("Kentish Town","Station"),["CONNECTION"], "FLOAT", "distance", _)
Below is the visualized result of the query:
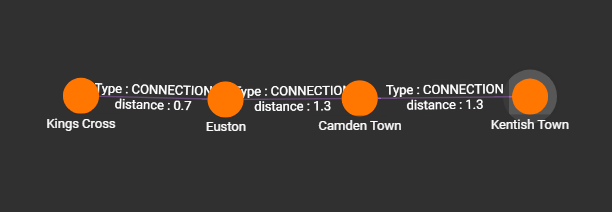